Loading Table with Cascading drop downs and Text boxes. When
User Click on Submit button only selected checked box row data store in the DB.
Step 1:- Put SCRIPT in the HTML Head tag
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$("#btnLoadData").click(function () {
//Reset table except header
$("#table2").find("tr:gt(0)").remove();
//Call LoadData Action
$.get("/demo/LoadData", function (response) {
//Loop data
$.each(response, function (i, k) {
var tRows = "<tr>"
tRows += "<td><input type='checkbox'
class='checkBox'></td>";
tRows += "<td>" + k.CustomerId + "</td>";
tRows += "<td>" + k.Name + "</td>";
tRows += "<td>" + k.Country + "</td>";
tRows += "<td><select
class='FirstDropDown'></select></td>";
tRows += "<td><select class='SecondDropDown'><option
value=''>Select</option></select></td>";
tRows += "<td><input type='text'
class='FirstTextBox'></td>";
tRows += "<td><input type='text'
class='SecondTextBox'></td>";
tRows += "</tr>";
//Append row to table
$("#table2").append(tRows);
});
//Call Category Action to bind
First DropDown
$.get("/demo/Category", function (response) {
//Set 1st option value 'blank' and
text 'select'
$(".FirstDropDown").append($('<option></option>').val('').text('Select'));
//Loop data
$.each(response, function (i, k) {
//Append option to FirstDropDown
class property
$(".FirstDropDown").append($('<option></option>').val(k.Id).text(k.Name));
});
});
});
});
//Create Change event for First
dropdown
$(document).on('change', '.FirstDropDown', function () {
//Get First DropDown selected value
var strVal = $(this).val();
//Get First DropDown closest 'tr'
var row = $(this).closest('tr');
//Find second DropDown from row
var secondDropDown = row.find('.SecondDropDown');
var secondTextBox = row.find('.SecondTextBox');
if (strVal == 1) {
secondTextBox.attr("disabled", "disabled");
}
else {
secondTextBox.removeAttr("disabled", "disabled");
}
//OR
var $item = $(this).parent().next().find('.SecondDropDown');
//Clear second DropDown before bind
secondDropDown.empty();
//Set 1st option value 'blank' and
text 'select'
secondDropDown.append($('<option></option>').val('').text("Select"));
//Call CategoryItems Action to bind
Second DropDown
$.get("/demo/CategoryItems", { "Id": strVal }, function(response) {
//Loop data
$.each(response, function (i, k) {
secondDropDown.append($('<option></option>').val(k.Id).text(k.Name));
//OR
//$item.append($('<option></option>').val(k.Id).text(k.Name));
});
});
});
//Click Submit button to Save Data
$("#btnSubmit").click(function () {
//Create json arrary Object
var jsonObj = [];
//Loop Checked Rows only
$("#table2
tr:has(input:checked)").each(function () {
//Create item Object
//var item = {};
//Add value to item Object
//item["CustomerId"] =
$(this).find('td:eq(1)').text();
//item["Name"] =
$(this).find('td:eq(2)').text();
//item["Country"] =
$(this).find('td:eq(3)').text();
//item["Category"] =
$(this).find('.FirstDropDown option:selected').text();
//item["CategoryItems"] =
$(this).find('.SecondDropDown option:selected').text();
//item["FirstTextBox"] =
$(this).find('.FirstTextBox').val();
//item["SecondTextBox"] =
$(this).find('.SecondTextBox').val();
//OR
//Add value to item Object
//item.CustomerId =
$(this).find('td:eq(1)').text();
//item.Name = $(this).find('td:eq(2)').text();
//item.Country =
$(this).find('td:eq(3)').text();
//item.Category =
$(this).find('.FirstDropDown option:selected').text();
//item.CategoryItems =
$(this).find('.SecondDropDown option:selected').text();
//item.FirstTextBox =
$(this).find('.FirstTextBox').val();
//item.SecondTextBox =
$(this).find('.SecondTextBox').val();
//OR
//Push item Object to json arrary
Object
//jsonObj.push(item);
//Push json arrary Object
jsonObj.push({
"CustomerId": parseInt($(this).find('td:eq(1)').text()),
"Name": $(this).find('td:eq(2)').text(),
"Country": $(this).find('td:eq(3)').text(),
"Category": $(this).find('.FirstDropDown option:selected').text(),
"CategoryItems": $(this).find('.SecondDropDown option:selected').text(),
"FirstTextBox": $(this).find('.FirstTextBox').val(),
"SecondTextBox": $(this).find('.SecondTextBox').val()
});
});
console.log(jsonObj);
//Call SaveToDB action function
$.ajax({
type: "POST",
url: "/demo/SaveToDB",
data: '{customer: ' + JSON.stringify(jsonObj) + '}',
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (response) {
alert(response.Msg);
},
failure: function (response) {
alert(response);
}
});
});
});
</script>
Step 2:- Put HTML in the HTML Body tag
<input id="btnLoadData" type="button" value="Load Data" />
<br />
<table id="table2" border="1" style="border-collapse: collapse;">
<tr>
<th>
Check
</th>
<th>
Customer Id
</th>
<th>
Name
</th>
<th>
Country
</th>
<th>
Category
</th>
<th>
Category Items
</th>
<th>
Textbox1
</th>
<th>
Textbox2
</th>
</tr>
</table>
<br />
<input id="btnSubmit" type="button" value="Submit" />
Step 3:- Create Customer.cs Class in Model folder
public class Customer
{
public int CustomerId { get; set; }
public string Name { get; set; }
public string Country { get; set; }
}
Step 4:- Create Category.cs Class in Model folder
public class Category
{
public int Id { get; set; }
public string Name { get; set; }
}
Step 5:- Create CategoryItem.cs Class in Model folder
public class CategoryItem
{
public int Id { get; set; }
public string Name { get; set; }
}
Step 6:- Create SaveData.cs Class in Model folder
public class SaveData
{
public int CustomerId { get; set; }
public string Name { get; set; }
public string Country { get; set; }
public string Category { get; set; }
public string CategoryItems { get; set; }
public string FirstTextBox { get; set; }
public string SecondTextBox { get; set; }
}
Step 7:- Copy Paste Action to DemoController.cs Class in Controllers folder
public ActionResult LoadData()
{
List<Customer> custList = new List<Customer>();
custList.Add(new Customer() { CustomerId = 1, Name = "Ram", Country = "India" });
custList.Add(new Customer() { CustomerId = 2, Name = "Shyam", Country = "India" });
custList.Add(new Customer() { CustomerId = 3, Name = "Ghanshyan", Country = "India" });
return Json(custList, JsonRequestBehavior.AllowGet);
}
public ActionResult Category()
{
List<Category> categoryList = new List<Category>();
categoryList.Add(new Category() { Id = 1, Name = "Fruit" });
categoryList.Add(new Category() { Id = 2, Name = "Veggie" });
return Json(categoryList, JsonRequestBehavior.AllowGet);
}
public ActionResult CategoryItems(int id)
{
List<CategoryItem> categoryItemList = new List<CategoryItem>();
categoryItemList.Add(new CategoryItem() { Id = 1, Name = "Red Apples" });
categoryItemList.Add(new CategoryItem() { Id = 1, Name ="Cherries" });
categoryItemList.Add(new CategoryItem() { Id = 1, Name ="Bananas" });
categoryItemList.Add(new CategoryItem() { Id = 2, Name ="Cauliflower" });
categoryItemList.Add(new CategoryItem() { Id = 2, Name = "Garlic"});
categoryItemList.Add(new CategoryItem() { Id = 2, Name = "Ginger"});
categoryItemList =
categoryItemList.Where(c => c.Id == id).ToList();
return Json(categoryItemList, JsonRequestBehavior.AllowGet);
}
public ActionResult SaveToDB(List<SaveData> customer)
{
string result = string.Empty;
//Add your DB Save code here
//Result "Success" or
"Fail"
result = "Success";
return Json(new { Msg = result }, JsonRequestBehavior.DenyGet);
}
Step 8:- Output
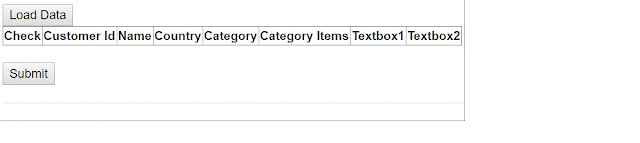